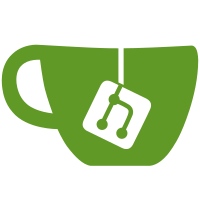
EROFS, like Squashfs, is a read-only file system. It can be used to store airootfs in an image file. Its advantage is the support for POSIX ACLs. EROFS downside is that currently it only supports LZ4 compression (LZMA support is not yet fully implemented). A difference from Squashfs is that, EROFS stores change time (ctime) not modification time (mtime). The reverse is true for Squashfs. Implements https://gitlab.archlinux.org/archlinux/archiso/-/issues/59
75 lines
2.5 KiB
Bash
75 lines
2.5 KiB
Bash
#!/bin/ash
|
|
#
|
|
# SPDX-License-Identifier: GPL-3.0-or-later
|
|
|
|
run_hook() {
|
|
# shellcheck disable=SC2154
|
|
# defined via initcpio's parse_cmdline()
|
|
if [ -n "${ip}" ] && [ -n "${archiso_http_srv}" ]; then
|
|
|
|
# booting with http is always copy-to-ram, so set here to make sure
|
|
# addresses are flushed and interface is set down
|
|
export copytoram="y"
|
|
|
|
archiso_http_srv=$(eval echo "${archiso_http_srv}")
|
|
[ -z "${archiso_http_spc}" ] && archiso_http_spc="75%"
|
|
|
|
export mount_handler="archiso_pxe_http_mount_handler"
|
|
fi
|
|
}
|
|
|
|
# Fetch a file with CURL
|
|
#
|
|
# $1 URL
|
|
# $2 Destination directory inside httpspace/${archisobasedir}
|
|
_curl_get() {
|
|
local _url="${1}"
|
|
local _dst="${2}"
|
|
|
|
msg ":: Downloading '${_url}'"
|
|
# shellcheck disable=SC2154
|
|
# defined via initcpio's parse_cmdline()
|
|
if ! curl -L -f -o "/run/archiso/httpspace/${archisobasedir}${_dst}/${_url##*/}" --create-dirs "${_url}"; then
|
|
echo "ERROR: Downloading '${_url}'"
|
|
echo " Falling back to interactive prompt"
|
|
echo " You can try to fix the problem manually, log out when you are finished"
|
|
launch_interactive_shell
|
|
fi
|
|
}
|
|
|
|
archiso_pxe_http_mount_handler () {
|
|
newroot="${1}"
|
|
local img_type="sfs"
|
|
|
|
msg ":: Mounting /run/archiso/httpspace (tmpfs) filesystem, size='${archiso_http_spc}'"
|
|
mkdir -p "/run/archiso/httpspace"
|
|
mount -t tmpfs -o size="${archiso_http_spc}",mode=0755 httpspace "/run/archiso/httpspace"
|
|
|
|
# shellcheck disable=SC2154
|
|
# defined via initcpio's parse_cmdline()
|
|
if ! curl -L -f -o /dev/null -s -r 0-0 "${archiso_http_srv}${archisobasedir}/${arch}/airootfs.sfs"; then
|
|
if curl -L -f -o /dev/null -s -r 0-0 "${archiso_http_srv}${archisobasedir}/${arch}/airootfs.erofs"; then
|
|
img_type="erofs"
|
|
fi
|
|
fi
|
|
_curl_get "${archiso_http_srv}${archisobasedir}/${arch}/airootfs.${img_type}" "/${arch}"
|
|
|
|
# shellcheck disable=SC2154
|
|
# defined via initcpio's parse_cmdline()
|
|
if [ "${checksum}" = "y" ]; then
|
|
_curl_get "${archiso_http_srv}${archisobasedir}/${arch}/airootfs.sha512" "/${arch}"
|
|
fi
|
|
# shellcheck disable=SC2154
|
|
# defined via initcpio's parse_cmdline()
|
|
if [ "${verify}" = "y" ]; then
|
|
_curl_get "${archiso_http_srv}${archisobasedir}/${arch}/airootfs.${img_type}.sig" "/${arch}"
|
|
fi
|
|
|
|
mkdir -p "/run/archiso/bootmnt"
|
|
mount -o bind /run/archiso/httpspace /run/archiso/bootmnt
|
|
|
|
archiso_mount_handler "${newroot}"
|
|
}
|
|
|
|
# vim: set ft=sh:
|